- Aniol Carbó
Web3 Ethereum Sign-In with Vottun APIs
Web3 Ethereum Sign-In allows users to log in to a website using their Ethereum wallet. This is possible through the use of digital signatures in the Ethereum blockchain. In this tutorial, we will show you how to implement a Web3 Ethereum Sign-In into a website based on the ERC-4361 standard.
For this tutorial, we will be using the Metamask wallet and the Vottun Web3 API.
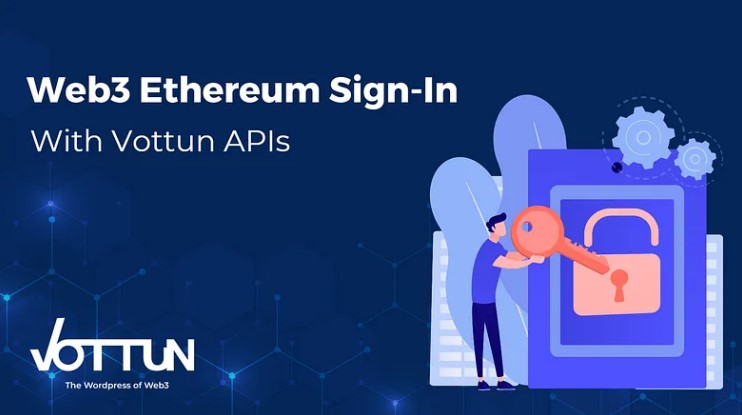
Prerequisites
Before you start developing, you need to have the following:
- Ethereum wallet (e.g. Metamask)
- “Ethers” library
- Vottun API credentials
The steps needed to achieve the implementation of the Web3 Login are:
- Implement a “Connect wallet” button for connecting a Metamask wallet.
- Once the user connects the wallet, make an API call to the Vottun Web3 API in order to obtain the log-in message.
- Trigger the “Sign” window in Metamask in order to make the user sign the message with his wallet.
- Once the user has signed the message, retrieve the signature and make an API call to the Vottun Web3 API in order to verify the signature validity.
How to get my credentials
Getting credentials is very easy. You have the option to get a 30 days trial at the url https://apis.vottun.tech/trial. Fill in the form and you will receive an email like the one below with your credentials ready to use.
In addition to the credentials, you also will receive the public key of a wallet that you can use in your tests (for security reasons, the private key is always kept on our servers).
This email will provide you with the JWT token (used as Bearer Authentication) and the Application ID, which is passed in another header. The detailed explanation of this configuration is explained further.
You will find more information about APIs at https://developers.vottun.tech.
Let’s dive into the implementation of each step:
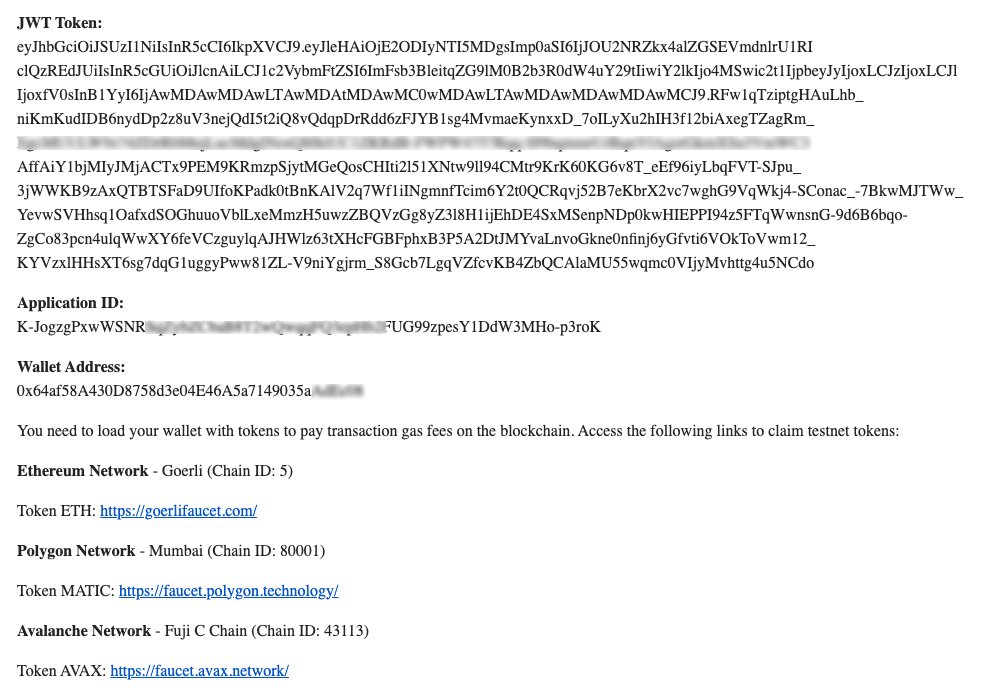
Step 1: Implement a "Connect wallet" button
The first step is to implement a “Connect wallet” button that will allow users to connect their Metamask wallet to your website. As Metamask is a browser extension, the interaction with its methods is performed through the `window.ethereum` object. If you are using a javascript/typescript framework, here’s the snippet for the “Connect Wallet” method:
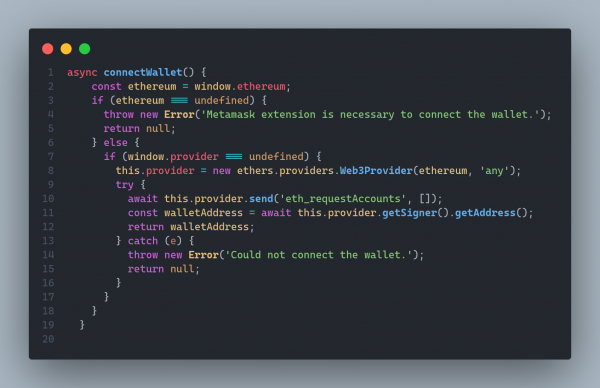
Step 2: Obtain the log-in message
The ERC-4361 standard defines a structured plaintext message that is signed by the user’s wallet, which generates a signature that can be validated in order to know if the user actually owns the connected wallet. As provided in the Ethereum official website, here is an example of this message:
vottun.tech wants you to sign in with your Ethereum account:
0xC02aaA39b223FE8D0A0e5C4F27eAD9083C756Cc2
I accept the Vottun Terms of Service: https://vottun.tech/tos
URI: https://vottun.tech/login
Version: 1
Chain ID: 1
Nonce: 32891756
Issued At: 2021-09-30T16:25:24Z
Resources:
- ipfs://bafybeiemxf5abjwjbikoz4mc3a3dla6ual3jsgpdr4cjr3oz3evfyavhwq/
- https://example.com/my-web2-claim.json
In order to obtain this message, the Vottun API provides a POST call that generates it with the correct format. This call expects two headers:
- An Authentication header, that must contain the Bearer token in it
- A “x-application-vk” header, that must contain the Application ID.
These two values are obtained at https://apis.vottun.tech/trial, following the steps described in the beginning. This call also expects a body with the following attributes:
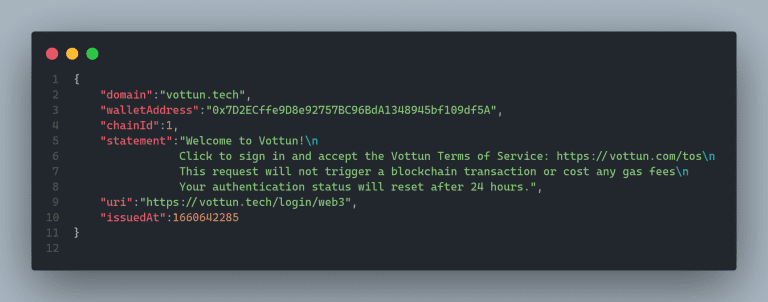
These are the mandatory fields required by the ERC-4361, and its meaning can be found in the official documentation (https://eips.ethereum.org/EIPS/eip-4361). The CURL snippet of this API call is:
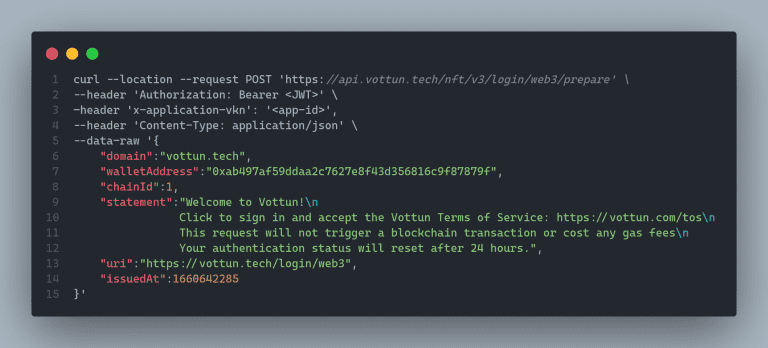
This endpoint returns a JSON with two attributes:
- Nonce: an identifier of this message, in order to validate it afterwards
- Message: the structured plaintext message that will be signed
Step 3: Sign the message
After obtaining the message, the wallet has to display a “Signature request” in order to make the user sign the message. This can be achieved through the “Ethers” library, using the “provider” object that we stored when connecting the wallet. If you are using a javascript/typescript framework, here’s the snippet for the “Sign Message” method:
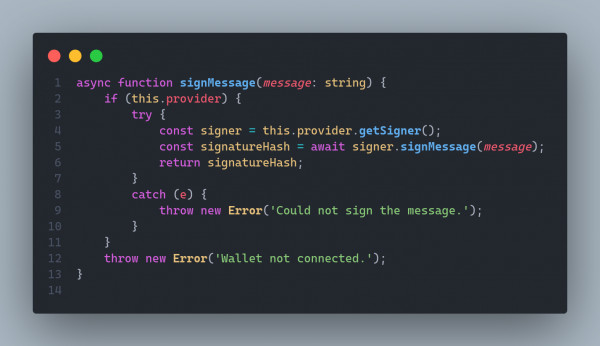
This method will trigger the “Signature Request” window of the installed wallet. Once the user accepts the signature, this method will return the hash that represents the performed signature.
Step 4: Verify the signature
The last step is verifying that the performed signature is valid, which means that the user actually owns the provided wallet address. The Vottun API provides a GET call that performs this verification:

The only two parameters (passed as query params) needed for this verification are:
- Nonce: Which was provided by the API itself in the message generation, used as an identifier
- Signature: Which is the signature hash returned by the `signMessage()` method.
The result of this API call is either a 200 OK status code if the signature is valid or a 403 Forbidden status code if the signature is invalid.
Conclusions
In conclusion, implementing a Web3 Ethereum Sign-In into a website based on the ERC-4361 standard can enhance the user experience by allowing users to log in to the website using their Ethereum wallet. This tutorial has shown developers how to implement a Web3 Ethereum Sign-In using the Metamask wallet and the Vottun Web3 API. By following the steps outlined in this tutorial, developers can enable their website to authenticate users using Ethereum digital signatures, which are secure and transparent. This also allows developers to create decentralized applications that leverage the full potential of the Ethereum blockchain without the need of a third-party authentication provider.